Use ES6 modules in the browser today with SystemJS
It's super frustrating that ES6 module syntax is officially part of the language specification, yet no browser supports it yet!
So most of the times, if you just want to use ES6 modules, you need to use something like Webpack or Browserify, adding one more step to do before you can actually start coding.
Of course, for production, you would want to bundle the files anyway, but is there no way to just quickly get started with development without all these steps?
Enter SystemJS
Luckily, these problems aren't new, so there are new tools that have appeared to help.
System.js is one of these tools. It is a module loader that, besides ES6, can also load global modules, AMD or CommonJS modules.
The nice part about it is that you don't need any extra build step to use it. Just include it in your index.html
and that's it.
Show me the code
We'll only have three files: two ES6 modules, one including the other, and one index.html
files to put them all together.
We'll include SystemJS using npm
.
Prepare some ES6 modules
First file, an Animal farm :D
// js/animal-farm.js
export const duck = {
quack: function() {
console.log('Quack quack')
}
};
export const dog = {
bark: function() {
console.log('Woof woof');
}
};
export const cat = {
meow: function() {
console.log('meow meow');
}
};
And the second, the main entry point:
// js/main.js
import { dog, cat } from 'animal-farm.js';
dog.bark();
cat.meow();
Download SystemJS and the Babel plugin for ES6
npm install systemjs sytemjs-plugin-babel
Include them in the index.html
<script src="node_modules/systemjs/dist/system.js"></script>
<script>
// Configure the SystemJS module loader
SystemJS.config({
// Tell it that our javascript files are in the /js folder
baseURL: '/js',
// Tell it to apply Babel to our JS files
transpiler: 'plugin-babel',
// Configure the Babel plugin - it's basically just telling it where to read the files from - in our case, they're in node_modules
map: {
'plugin-babel': '../node_modules/systemjs-plugin-babel/plugin-babel.js',
'systemjs-babel-build': '../node_modules/systemjs-plugin-babel/systemjs-babel-browser.js'
},
});
// Now that the configuration is complete, we can include our main file! (/js/main.js)
SystemJS.import('main.js');
</script>
Open the browser
Aaaand that's it, you're application is running! You should see the console.log
messages in the DevTools Console.
But wait, what about debugging?
You'll notice that even if the code is run directly in the browser, transpilation still occurs, except that it's at runtime.
However, sourcemaps work out of the box, so you can still continue to debug your code normally. The only change is that in the sources folder you will see both files: original and transpiled:
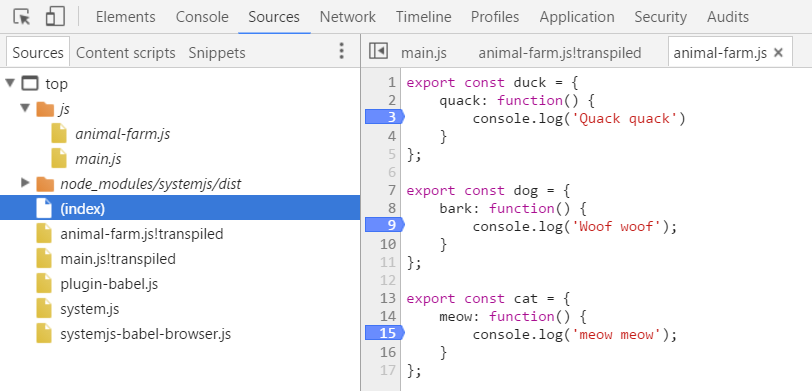
Comments ()